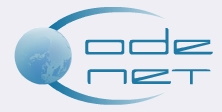
Autoindenting PHP Code
Introduction
PHP code uses {} symbols to group pieces of code such as branches and loops. This code makes use of that by checking for the existence of them in each line. If it find a { then there should be a positive indent of the next line. If it finds a } then there should be a negative indent of the current line.
How to use the code
The code requires a reference to the "Microsoft Scripting Runtime" in order to make use of the FileSystemObject to read in and write out the file. Currently it is also written for Excel in that is uses GetOpenFileName to obtain the file to parse, this could easily be modified for VB to use a common dialog.
How it works
The code uses 1 Sub and 2 helper functions and uses a global variable to store the current indent level. When it starts it sets the indent to 0 and opens the file. It then loops through each line in the file, parses it using the FormatPHPLine function and stores it in an array. It then loops through the array to write the lines back out to the file
The FormatPHPLine checks for the occurrence of { and } to detremine if a positive or negative indent is required. If a negative is found it is applied strightaway i.e. the global variable is decreased by 1. The required indent is then created using the indent function. Finally the global variable is increased by 1 if a positive indent was found.
Improvements
There is much scope for improvement, such as, allowing for multiple occurrences on one line or making sure only code not comments are searched which I may add in the future.
The Full Code
Dim intIndent As Long Sub FormatPHP() Dim strFile As String intIndent = 0 strFile = Application.GetOpenFilename("PHP Files (*.php),*.php") If strFile = "False" Then Exit Sub Dim fso As Scripting.FileSystemObject Set fso = New Scripting.FileSystemObject Dim ts As Scripting.TextStream Dim strText() As String Dim x As Integer x = 0 Set ts = fso.OpenTextFile(strFile, ForReading, FALSE) While Not ts.AtEndOfStream x = x + 1 ReDim Preserve strText(1 To x) strText(x) = FormatPHPLine(ts.ReadLine) Wend ts.Close Set ts = fso.OpenTextFile(strFile, ForWriting, FALSE) For i = 1 To x ts.WriteLine strText(i) Next i ts.Close MsgBox "Done!" End Sub Function FormatPHPLine(ByVal strPHP As String) As String 'check For { And } To determine indent 'if { Then positive indent 'if } Then negative Dim pos As Boolean, neg As Boolean If InStr(1, strPHP, "{") > 0 Then pos = TRUE End If If InStr(1, strPHP, "}") > 0 Then neg = TRUE End If 'apply negative indent If neg Then intIndent = Application.WorksheetFunction.Max(0, intIndent - 1) End If strPHP = indent(strPHP) 'apply +ve indent If pos Then intIndent = intIndent + 1 End If FormatPHPLine = strPHP End Function Function indent(ByVal y As String) As String y = Trim$(y) If intIndent > 0 Then For i = 1 To intIndent indent = indent & vbTab Next i indent = indent & y Else indent = y End If End Function