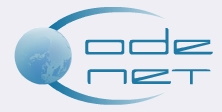
Use of parentheses
The rules of thumb:
- Always use parentheses when you use the Call statement:
Call Highlight(txtFirstName)
- Always use parentheses when calling a function and capturing the return value:
Response = MsgBox("Do you want to continue?", buttons:=vbYesNo)
- Don't use parentheses when calling a sub/function without "Call"
Highlight txtFirstName
- Don't use parentheses when calling a function but not capturing the return value:
MsgBox prompt:="Hello world", title:="Say hi"
If you put parentheses where there should be none, it can sometimes lead to subtle errors that are difficult to understand. For example, say you have a procedure that takes a textbox object as an argument:
Public Sub Highlight(ByVal xText As Object) xText.SelStart = 0 xText.SelLength = Len(xText.Text) xText.SetFocus End Sub
You try calling it like this, and it doesn't work:
Highlight (txtFirstName)You can see that VBE has inserted a space before the parentheses. That's a sign that parentheses are not needed.
In a situation like this, where the sub has only one argument, adding parentheses in the wrong place does not cause a compile error. Instead, VBE interprets the parentheses as being around the argument, and not the argument list. In doing that, it turns a reference to the textbox object into a reference to the object's default property. So in this case, what gets passed to the sub is txtFirstName.Text and not a reference to the object itself.
The following example should emphasise this point:
Private Sub Form_Load() Dim tb As TextBox Set tb = Me.Text1 Tester tb Tester (tb) Unload Me End Sub Sub Tester(v As Variant) MsgBox TypeName(v) End Sub