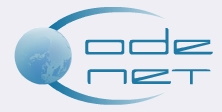
Sending an email with an attachment with PHP
The code below will upload an attachment and email it as long as it's a zip file. You could easily modify it to take any other files though. It relies on the 'file' type inputbox to be named 'FileUpload'. e.g.
<input type="file" name="FileUpload" size="20">
//upload file and send email through
$tmpfile=$HTTP_POST_FILES['FileUpload']['tmp_name'];
$filename=stripslashes($HTTP_POST_FILES['FileUpload']['name']);
$filesize=$HTTP_POST_FILES['FileUpload']['size'];
$filetype=$HTTP_POST_FILES['FileUpload']['type'];
$attach=true;
if (($tmpfile=='none')||($filesize==0)) {
$attach=false;
}
if (($filetype!='application/x-zip-compressed')&&($filetype!='application/zip')) {
$errors++;
$err_msgs[$errors-1]="Error: Only zip files can be uploaded.".$filetype." test";
}
if ($filesize>2000) {
$errors++;
$err_msgs[$errors-1]="Error: Files must be less than 2MB.";
}
if (!is_uploaded_file($tmpfile)) {
$errors++;
$err_msgs[$errors-1]="Error uploading file.";
}
if($errors==0) {
//create email and send
if ($attach) {
//sort the file
$file = fopen($tmpfile,'rb');
$data = fread($file,filesize($tmpfile));
fclose($file);
// Base64 encode the file data
$data = chunk_split(base64_encode($data));
}
$mime_boundary = md5(uniqid(time()));
$message="test";
//headers
$headers .= "From: Name <emailaddress>n";
$headers .= "MIME-Version: 1.0nContent-Type: multipart/mixed;boundary="".$mime_boundary."";n";
$headers .= "charset="iso-8859-1"nContent-Transfer-Encoding:7bitnn";
//start message
$headers .= "This is a multi-part message in MIME format.n";
$headers .= "n";
$headers .= "--".$mime_boundary."n";
//now text message
$headers .= "Content-Type: text/plain; charset="iso-8859-1"n";
$headers .= "Content-Transfer-Encoding: 7bitnn";
$headers .= $message."nn";
if ($attach) {
//now the file
$headers .= "--".$mime_boundary."n";
$headers .= "Content-Type: {".$filetype."}; name="".$filename.""n";
$headers .= "Content-Transfer-Encoding: base64n";
$headers .= "Content-Disposition: attachmentnn";
$headers .= $data;
}
$headers .= "--".$mime_boundary."--rn";
// Send the message
$ok = @mail("emailaddress", "Subject", $message, $headers);
if (!$ok) {
$errors++;
$err_msgs[$errors-1]="Error: Unable to send email, please try again.";
}
}